In one of my previous blog post, i had written about how to have a custom date filter column in Kendo UI grid but the JavaScript way. You can read about it here. Video version of that blog post is available here. In this blog post i will showcase how to achieve the same scenario but using ASP.NET MVC Wrapper.
Prerequisite:
In order to follow along with this blog post, you will need to have downloaded our product “UI for ASP.NET MVC“. We have a 30 day trial of the product for evaluation purpose. You can download the trial from the product page here. Rest of this post, i am assuming you have installed the product and you are ready to follow along this post.
Northwind OData Service:
Instead of hand crafting a Web API or a MVC based JSON end point to read data, i will be using a demo OData service available at www.odata.org. It will be a Northwind OData Service and we will be using the Order table for this exercise. The service is available here.
Create New Project:
When you install Telerik “UI for ASP.NET MVC” product, one of the things we integrate with Visual Studio is that of Project Templates. We install a “Telerik C# ASP.NET MVC Application” project template. As the name goes, this project template will create a new ASP.NET MVC template and add reference to Kendo UI class library and also add reference to Kendo UI JavaScript/Stylesheets.

Fig 1. Telerik C# ASP.NET MVC Application
Kendo UI Grid Creation:
First up, lets open index.cshtml file under Views > Home folder and instantiate a Kendo UI Grid. Here is the code snippet for instantiating the Kendo UI Grid using our MVC wrappers:
@(Html.Kendo().Grid<Order>() | |
.Name("grid") | |
) |
I have created a model class by the name Order. This class contains the properties i am interested to show in the grid and correspond to appropriate column on the service.
Bind Northwind OData Service to Grid:
Next, we will bind the Northwind Orders OData Service as the data source for the grid. The Kendo UI Grid wrapper exposes Datasource() helper method and we will use this to configure the data source for the grid. Here is the code snippet to configure data source:
@(Html.Kendo().Grid<Order>() | |
.Name("grid") | |
.DataSource(source => | |
source.Custom() | |
.Type("odata-v4") | |
.Transport(transport => { | |
transport.Read(read => | |
{ | |
read.Url("http://services.odata.org/V4/Northwind/Northwind.svc/Orders"); | |
}); | |
}) | |
.PageSize(10) | |
.ServerPaging(true) | |
.ServerFiltering(true) | |
) | |
.Pageable() | |
.Sortable() | |
.Selectable(sortable => sortable.Mode(GridSelectionMode.Single)) | |
.Columns(columns => | |
{ | |
columns.Bound(c => c.OrderID).Title("Order"); | |
columns.Bound(c => c.CustomerID).Title("Customer"); | |
columns.Bound(c => c.ShipName).Title("Shipper"); | |
columns.Bound(c => c.ShipCity).Title("City"); | |
columns.Bound(c => c.ShipCountry).Title("Country"); | |
columns.Bound(c => c.OrderDate).Title("Ordered"); | |
columns.Bound(c => c.RequiredDate).Title("Required"); | |
columns.Bound(c => c.ShippedDate).Title("Shipped"); | |
}) | |
) |
While configuring the data source, we perform the following things:
- Set Data Source Type – in this case we have set to “odata-v4”
- Set the Read URL – the end point which will be invoked by grid data source to read data
- Set Page Size
- Set Server Side Paging to true
- Set Server Side Filtering to true
Apart from the data source configuration we also configure the following things on the Grid itself:
- Pageable – let the grid know that it is pageable. We will get the paging bar.
- Sortable – let the grid know that it is sortable. Column headers will be made sortable header
- Selectable – let the grid know that the rows can be selected on a mouse click.
- Columns – let the grid know which columns it should show in the grid
Below is a screenshot of the grid that is rendered as per the configuration we have done so far:

Fig 2. Kendo UI Grid
Enabling Filter on the columns:
To enable filtering on the grid columns, we just have to use Filterable() helper method. Filterable() helper method is set on the grid itself. Here is the code snippet to enable the filter:
@(Html.Kendo().Grid<Order>() | |
.Name("grid") | |
@*...*@ | |
.Filterable() | |
@*...*@ | |
) |
Let see the output of the grid after enabling filter:

Fig 3. Kendo UI Grid with Filters
But we 2 problems with the grid now. They are:
- All columns have got the filter now. We want filter only on Ordered column.
- When we check the operators available for Date column we see all the options supported out of the box. Instead what we need is “From Date” & “To Date”.
Custom Date Filter Options:
In order to solve the above problem we need to perform the following actions:
- Set Filterable to false on columns where we don’t need filters
- On the grid Filterable() we will need to let the grid know that we don’t need all the options available out of the box but have only 2 options which is “Greater Than Or Equal To” and “Less Than Or Equal To”
Here is the code snippet which will solve the above filter problem:
@(Html.Kendo().Grid<Order>() | |
.Name("grid") | |
@*...*@ | |
.Filterable(filterable => filterable.Operators(operators => | |
{ | |
operators.ForDate(options => | |
{ | |
options.Clear(); | |
options.IsGreaterThanOrEqualTo("From Date"); | |
options.IsLessThanOrEqualTo("To Date"); | |
}); | |
})) | |
.Columns(columns => | |
{ | |
columns.Bound(c => c.OrderID).Title("Order").Filterable(false); | |
columns.Bound(c => c.CustomerID).Title("Customer").Filterable(false); | |
columns.Bound(c => c.ShipName).Title("Shipper").Filterable(false); | |
columns.Bound(c => c.ShipCity).Title("City").Filterable(false); | |
columns.Bound(c => c.ShipCountry).Title("Country").Filterable(false); | |
columns.Bound(c => c.OrderDate).Title("Ordered").Format("{0:MMM dd yyyy}"); | |
columns.Bound(c => c.RequiredDate).Title("Required").Filterable(false).Format("{0:MMM dd yyyy}"); | |
columns.Bound(c => c.ShippedDate).Title("Shipped").Filterable(false).Format("{0:MMM dd yyyy}"); | |
}) | |
) |
Notice how we have enabled operators for “Date “data type. Kendo UI grid wrapper makes it easier to customize the gird according to our needs. Here is the output of the above code:
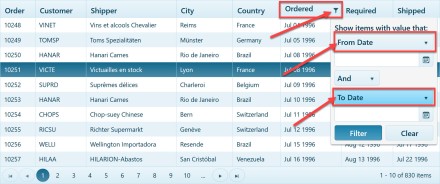
Fig 4. Kendo UI Grid with Custom Date Filter
That’s it – we have customized the Kendo UI Grid column filtering according to our needs. We have provided a custom options for date column filter in Kendo UI Grid.
In this blog post i showcases how to work with our Kendo UI MVC Wrappers to instantiate a grid and customize a column filter. Hope this helps you in your project, if you have a similar requirement.
Till next time – Happy Coding !
Pingback: Video: How to add custom Date filter column in Kendo UI Grid using ASP.NET MVC Wrapper | Telerik Helper - Helping Ninja Technologists