In this post we will walkthrough on consuming WCF REST Service in Kendo UI Mobile. We may consider this post is divided in two parts. In part 1, we will see the way to create REST based WCF service and in part2 of this post we will consume that REST Service in Kendo UI mobile.

Creating WCF REST Service
If you are new to WCF and wondering how to create WCF REST Service .Please read many articles on WCF REST Service here .
To get it started quickly fire visual studio and create a new project. From WCF tab select WCF Service Application project template.

Delete all the codes generated from both the IService1.cs and Service1.svc.cs file.
Although in this post we are not going to fetch and data from database. We will return C sharp collection as data from WCF REST Service. However we can use any methodologies to create data model and expose data from data model as service. To make it simple we are going to work with following business object.
public class Bloggers
{
[DataMember]
public string BloggerID { get; set; }
[DataMember]
public string Name { get; set; }
[DataMember]
public string Technology { get; set; }
}
Define service as following
[ServiceContract]
public interface IService1
{
[OperationContract]
[WebGet(UriTemplate = "/GetBloggers",
ResponseFormat = WebMessageFormat.Json,
RequestFormat = WebMessageFormat.Json)]
List<Bloggers> GetBloggers();
}
If you notice we have explicitly set the Request and Response message format as Json. Service is implemented as following. There is not much fancy about the service implementation, it is simply creating collection of business object and returning.
using System.Collections.Generic;
namespace RESTServiceForKendo
{
public class Service1 : IService1
{
public List<Bloggers> GetBloggers()
{
List<Bloggers> lstBloggers = new List<Bloggers>()
{
new Bloggers { BloggerID = "1", Name= "Jesse Liberty ", Technology = "XAML"},
new Bloggers { BloggerID = "2" , Name = "Michael Crump" , Technology = "XAML"},
new Bloggers { BloggerID = "3" , Name = "Chris Eargel" , Technology = "C Sharp"},
new Bloggers { BloggerID = "4" , Name = "Chris Sells" , Technology = "All"},
new Bloggers { BloggerID = "5" , Name = "John Bristowe" , Technology = "Web"},
new Bloggers { BloggerID = "6" , Name = "Todd Anglin " , Technology = "KendoUI"},
new Bloggers { BloggerID = "7", Name= "John Papa ", Technology = "XAML"},
new Bloggers { BloggerID = "8" , Name = "Glen Block" , Technology = "REST"},
new Bloggers { BloggerID = "9" , Name = "Lohith " , Technology = "Telerik"},
new Bloggers { BloggerID = "10" , Name = "Scott Hanselman " , Technology = "All"},
new Bloggers { BloggerID = "11" , Name = "Debugmode" , Technology = "Kendo"},
new Bloggers { BloggerID = "12" , Name = "Pinal Dave " , Technology = "SQL Server"},
new Bloggers { BloggerID = "12" , Name = "Julie Lerman" , Technology = "Entity Framework"}
};
return lstBloggers;
}
}
}
Next we need to configure Endpoint. Rather than creating Endpoint we are going to use factory class. Class System.ServiceModel.Activation.WebServiceHostFactory enables REST Endpoint on WCF Service. To set factory class right click on Service1.svc and click on view markup. In markup add factory class as following.
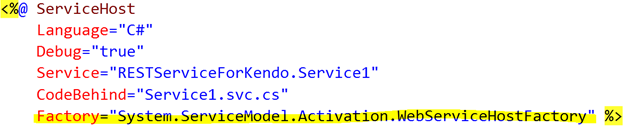
After configuring factory class we are all set with WCF REST Service. To test the service, run the service in browser. We should be getting Bloggers information as JSON data in browser.

Once you get data in browser (if you are using IE then you will be prompted to save returned data), we are all set to consume this data in Kendo UI Mobile.
Consuming in Kendo UI Mobile
We will consume JSON data from WCF REST service step. If you are using Visual Studio for Kendo UI development then you may find below post useful
Setting Development Environment in Visual Studio 2010 using NuGet for KendoUI
To proceed with follow the following steps
Step 1
Very first add the required references to work with Kendo UI Mobile. You need to add reference in head section of the page.
<head>
<title></title>
<meta charset="utf-8" />
<script src="kendo/js/jquery.min.js"></script>
<script src="kendo/js/kendo.mobile.min.js"></script>
<link href="kendo/styles/kendo.mobile.all.min.css" rel="stylesheet" />
</head>
After adding references we need to initialize the mobile. To do this adds below script just before the closing body tag.
<script>
var app = new kendo.mobile.Application(document.body, { transition: "slide", layout: "pageLayOut" });
</script>
</body>
You can see that layout and transition has been set. We will create layout in next step.
Step 2
After adding reference we need to create layout of the application. In layout we want two buttons in bottom tabstrip and a back button on the top. Layout can be created as following
<div data-role="layout" data-id="pageLayOut">
<header data-role="header">
<div data-role="navbar">
<a data-role="backbutton" data-align="left">Back</a>
<span data-role="view-title"></span>
</div>
</header>
<div data-role="footer">
<div data-role="tabstrip">
<a href="#homeView" data-icon="home">Home</a>
<a href="#bloggerView" data-icon="action">Bloggers</a>
</div>
</div>
</div>
Step 3
Next we need to create different views. As you notice from layout that we need to create two views. One is homeView and other bloggerView. In homeView we will display a message.
homeView is as following
<div data-role="view" id="homeView" id="homeView" data-title="Bloggers">
<h1>Welcome!</h1>
<p>
In this App we will fetch Bloggers information from WCF REST Service
</p>
</div>
Step 4
Now we need to create bloggerView. In this view we will put a Kendo UI Mobile ListView control. All the data returned from service will be bind to this ListView.
<div data-role="view" id="bloggerView" data-title="Bloggers" data-show="showBloggers">
<ul id="bloggerList"
data-source="bloggersData"
data-endlessScroll="true"
data-template="bloggersTemplate"
data-role="listview"
data-style="inset">
</ul>
</div>
There are couples of important points worth discussing about above code. In above listview we are setting data-source and data-template as following

So we need to
- Create data-source bloggersData from the returned JSON data from the WCF REST Service (step5)
- Create data-template bloggersTemplate (step4)
Step 4
Data Template allows us to render data as we want. It is used to format the displaying of data. A template can be created as following. We are creating a Kendo UI list view link button and displaying Name and Technology of the blogger. Id of this template is set as data-template property of list bloggerList.
<script id="bloggersTemplate" type="text/x-kendo-template">
<a href="\#BloggerDetailsView" class="km-listview-link" data-role="listview-link">
<h2>#=data.Name#</h2>
<h4>#=data.Technology#</h4>
</a>
</script>
Step 5
Now we need to create datasource. We are going to create Kendo UI DataSource reading JSON data from the service.

In Kendo UI DataSource configuration, read attribute has been set to the URL of the WCF REST Service. Since service is retuning JSON data hence Accept type is as application/json.
Finally in data-show method of view we will fetch data from the DataSource. If you notice data-show attribute of bloggerView is set to showBloggers.

Putting all the discussion together DataSource can be created and data from that can be fetched as shown in below code
var bloggersData;
bloggersData = new kendo.data.DataSource(
{
transport: {
read: {
url: "http://localhost:56863/Service1.svc/getBloggers",
data: {
Accept: "application/json"
}
}
}
});
function showBloggers(e) {
bloggersData.fetch();
Now we are all set to run the application. On running we should be getting Bloggers data as following
Home View
![clip_image001[6] clip_image001[6]](https://telerikhelper.files.wordpress.com/2012/07/clip_image0016_thumb.png?w=294&h=543)
Blogger View

In this way you can consumed a JSON based WCF REST Service in Kendo UI mobile. I hope you find this post useful. Thanks for reading.