We get frequent requests from customers wanting to build a document using a template with header and footer and just including text in the Word document using ASP.NET Core.
We can achieve this using the Telerik WordsProcessing library. In this blog post, we will explore how.
You can do this by importing the document template with the DocxFormatProvider (if the template is a DOCX document or with any other of the supported by the WordsProcessing format providers) into a RadFlowDocument, edit its content using the RadFlowDocumentEditor and export it with the appropriate format provider.
First, you need to to install Telerik.Documents.Flow NuGet package see below picture where I am using Trial version of Telerik.

Add Template.docx file in root directory with header and footer.
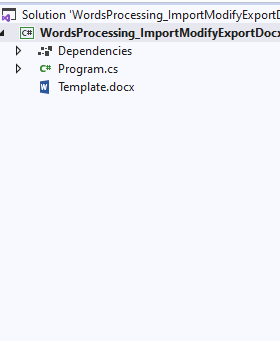
In Program.cs file
Step 1 : Add below mentioned namespaces :
using System.Diagnostics;
using Telerik.Windows.Documents.Flow.FormatProviders.Docx;
using Telerik.Windows.Documents.Flow.Model;
using Telerik.Windows.Documents.Flow.Model.Editing;
Step 2 : Create RadFlowDocument object.
RadFlowDocument document;
Step 3 : And add the DocxFormatProvider for importing the DOCX Template in RadFlowDocument object “document”.
DocxFormatProvider provider = new DocxFormatProvider();
string templatePath = "Template.docx";
using (Stream input = File.OpenRead(templatePath))
{
document = provider.Import(input);
}
Step 4 : Now add the RadFlowDocumentEditor for editing the RadFlowDocument with providing it’s object. and with the RadFlowDocumentEditor object “editor” we are inserting the text.
RadFlowDocumentEditor editor = new RadFlowDocumentEditor(document);
editor.InsertText("Telerik WordsProcessing Library");
Step 5 : Now Define the Output of Document path. and export the document using above mentioned RadFlowDocumentEditor object “provider”.
string outputDocumentPath = "Output.docx";
using (Stream output = File.OpenWrite(outputDocumentPath))
{
provider.Export(document, output);
}
Step 6 : And I am opening the file after exporting using below line of code you can also remove that if you don’t want to open file.
Process.Start(new ProcessStartInfo() { FileName = outputDocumentPath, UseShellExecute = true });
The whole program look likes below :

Now you can get the Word Document File with name of Output.docx.
And for more information about the RadFlowDocumentEditor you can find in the RadFlowDocumentEditor help article.
If you are new to this library, I would suggest starting with the Getting Started help article and checking the WordsProcessing`s Cross-Platform Support topic.